Get data from the Hamburg Urban Data Portal using WFS
Learn how to access vector data from the Hamburg Urban Data Portal with WFS
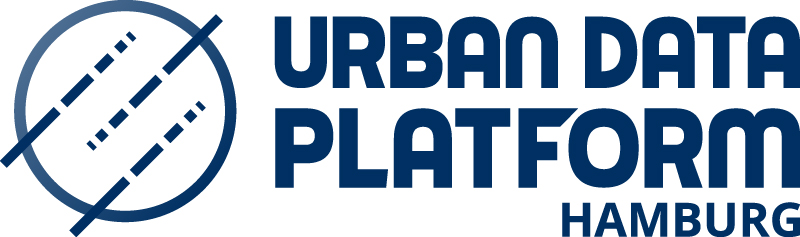
Introduction¶
The Hamburg Urban Data Platform¶
The Hamburg Urban Data Platform (UDP_HH) is a central component of the city’s digital transformation strategy, serving as a comprehensive hub for collecting, integrating, and disseminating urban data. Developed by the State Office for Geoinformation and Surveying (LGV) in collaboration with the CityScienceLab at HafenCity University, the platform offers standardized interfaces and real-time access to a wide array of datasets, including traffic counts, environmental measurements, and infrastructure details. These datasets are accessible to public authorities, businesses, researchers, and citizens, facilitating data-driven decision-making and fostering innovation. The platform’s user-friendly interface, known as the UDP Cockpit, allows users to explore and visualize data interactively, supporting various applications from urban planning to environmental monitoring. By promoting transparency and interoperability, the Urban Data Platform plays a pivotal role in enhancing Hamburg’s status as a smart city.
Purpose of this Jupyter Notebook¶
This jupyter notebook shows how to access data from the Hamburg Urban Data portal using WFS. In this example, we get:
- Data from the Hamburg Urban Data Portal Hochwasserschutzlinie im Land Bremen into a geopandas data frame
- Save the data into geosjon with epsg:4326 projection for further usage for instance in JupyterGIS.
pip install jupyterlab_myst geopandas geojson matplotlib
Import Python Packages¶
import os
from io import BytesIO # Import BytesIO
import geopandas as gpd
import requests
# WFS URL
wfs_url: str = (
"https://geoportal.saarland.de/arcgis/services/Internet/Hochwasser_WFS/MapServer/WFSServer?&request=GetCapabilities&VERSION=1.1.0&SERVICE=WFS"
)
# Feature type
feature_typename: str = "Hochwasser_WFS:Flaeche100_generalisiert"
# Output file name (Geojson)
filename: str = "HQ100_Flaeche100_generalisiert_4326.geojson"
Get data from the Hamburg Urban Data Portal using WFS¶
# Specify parameters
params = {
"service": "WFS",
"request": "GetFeature",
"typeName": feature_typename,
}
try:
# Fetch data
r = requests.get(wfs_url, params=params)
r.raise_for_status() # Check for HTTP errors
# Read GML directly into GeoDataFrame
gml_data = BytesIO(r.content)
data = gpd.read_file(gml_data, driver="GML")
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
except Exception as e:
print(f"Failed to parse GML: {e}")
Quick visualisation¶
data.plot()
Save to geojson with WSG84 (epsg:4326) projection¶
if os.path.exists(filename):
print("Skipped creation: file already exists.")
else:
data.to_crs(4326).to_file(filename, encoding="utf-8")